C++ How to Read in a File and Cout It
What is file handling in C++?
Files shop data permanently in a storage device. With file handling, the output from a program tin be stored in a file. Various operations can exist performed on the data while in the file.
A stream is an abstraction of a device where input/output operations are performed. Yous tin correspond a stream as either a destination or a source of characters of indefinite length. This will be determined by their usage. C++ provides you with a library that comes with methods for file handling. Let united states hash out information technology.
In this c++ tutorial, yous will learn:
- What is file treatment in C++?
- The fstream Library
- How to Open Files
- How to Shut Files
- How to Write to Files
- How to Read from Files
The fstream Library
The fstream library provides C++ programmers with three classes for working with files. These classes include:
- ofstream– This class represents an output stream. It'due south used for creating files and writing information to files.
- ifstream– This class represents an input stream. It's used for reading data from information files.
- fstream– This class generally represents a file stream. It comes with ofstream/ifstream capabilities. This means it's capable of creating files, writing to files, reading from information files.
The post-obit prototype makes it simple to empathize:
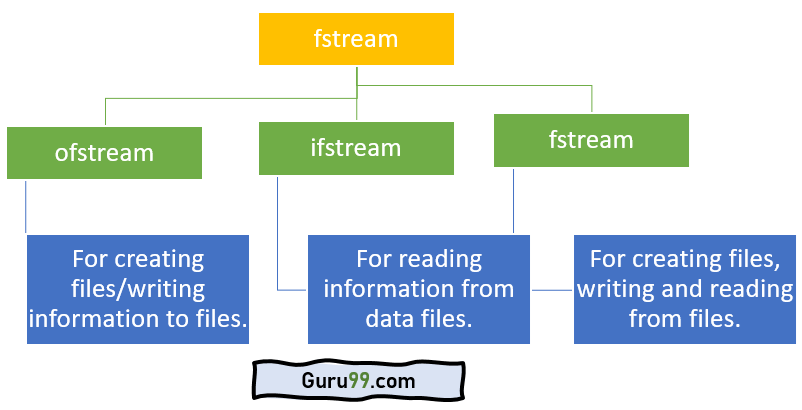
To employ the above classes of the fstream library, yous must include it in your programme as a header file. Of class, y'all will apply the #include preprocessor directive. Yous must as well include the iostream header file.
How to Open Files
Before performing whatever operation on a file, you must first open it. If you demand to write to the file, open up it using fstream or ofstream objects. If you only need to read from the file, open it using the ifstream object.
The iii objects, that is, fstream, ofstream, and ifstream, have the open() office defined in them. The office takes this syntax:
open up (file_name, fashion);
- The file_name parameter denotes the name of the file to open.
- The mode parameter is optional. It can take any of the following values:
Value | Description |
---|---|
ios:: app | The Suspend mode. The output sent to the file is appended to it. |
ios::ate | Information technology opens the file for the output so moves the read and write control to file'southward terminate. |
ios::in | Information technology opens the file for a read. |
ios::out | It opens the file for a write. |
ios::trunc | If a file exists, the file elements should be truncated prior to its opening. |
It is possible to employ two modes at the aforementioned time. You combine them using the | (OR) operator.
Instance one:
#include <iostream> #include <fstream> using namespace std; int main() { fstream my_file; my_file.open("my_file", ios::out); if (!my_file) { cout << "File non created!"; } else { cout << "File created successfully!"; my_file.close(); } return 0; }
Output:
Here is a screenshot of the code:
Lawmaking Explanation:
- Include the iostream header file in the plan to use its functions.
- Include the fstream header file in the program to use its classes.
- Include the std namespace in our lawmaking to utilize its classes without calling information technology.
- Telephone call the main() function. The program logic should get inside its trunk.
- Create an object of the fstream form and give information technology the proper noun my_file.
- Apply the open() function on the above object to create a new file. The out mode allows us to write into the file.
- Use if statement to check whether file cosmos failed.
- Message to print on the console if the file was non created.
- End of the trunk of if statement.
- Utilize an else argument to state what to do if the file was created.
- Bulletin to impress on the console if the file was created.
- Employ the close() function on the object to close the file.
- Cease of the body of the else statement.
- The program must return value if information technology completes successfully.
- End of the main() function torso.
How to Close Files
Once a C++ program terminates, it automatically
- flushes the streams
- releases the allocated memory
- closes opened files.
However, as a programmer, you should acquire to close open files before the program terminates.
The fstream, ofstream, and ifstream objects have the shut() part for closing files. The function takes this syntax:
void close();
How to Write to Files
You can write to file right from your C++ plan. You employ stream insertion operator (<<) for this. The text to be written to the file should be enclosed within double-quotes.
Allow usa demonstrate this.
Example two:
#include <iostream> #include <fstream> using namespace std; int principal() { fstream my_file; my_file.open("my_file.txt", ios::out); if (!my_file) { cout << "File not created!"; } else { cout << "File created successfully!"; my_file << "Guru99"; my_file.close(); } return 0; }
Output:
Here is a screenshot of the code:
Code Explanation:
- Include the iostream header file in the program to use its functions.
- Include the fstream header file in the program to utilize its classes.
- Include the std namespace in the program to use its classes without calling it.
- Call the chief() function. The program logic should be added within the body of this role.
- Create an example of the fstream class and give information technology the name my_file.
- Apply the open up() function to create a new file named my_file.txt. The file volition be opened in the out mode for writing into information technology.
- Use an if statement to cheque whether the file has non been opened.
- Text to print on the panel if the file is not opened.
- Cease of the body of the if argument.
- Use an else statement to country what to do if the file was created.
- Text to impress on the console if the file was created.
- Write some text to the created file.
- Use the close() function to shut the file.
- Finish of the trunk of the else statement.
- The plan must render value upon successful completion.
- End of the torso of the main() office.
How to Read from Files
Yous can read information from files into your C++ program. This is possible using stream extraction operator (>>). You employ the operator in the same way you use it to read user input from the keyboard. However, instead of using the cin object, you use the ifstream/ fstream object.
Example iii:
#include <iostream> #include <fstream> using namespace std; int main() { fstream my_file; my_file.open("my_file.txt", ios::in); if (!my_file) { cout << "No such file"; } else { char ch; while (one) { my_file >> ch; if (my_file.eof()) pause; cout << ch; } } my_file.close(); return 0; }
Output:
No such file
Here is a screenshot of the code:
Code Explanation:
- Include the iostream header file in the plan to use its functions.
- Include the fstream header file in the plan to use its classes.
- Include the std namespace in the program to use its classes without calling information technology.
- Call the main() function. The program logic should be added within the body of this function.
- Create an instance of the fstream course and requite information technology the name my_file.
- Use the open up() function to create a new file named my_file.txt. The file will be opened in the in mode for reading from it.
- Apply an if statement to bank check whether the file does not exist.
- Text to print on the console if the file is not institute.
- Cease of the body of the if statement.
- Apply an else argument to country what to do if the file is constitute.
- Create a char variable named ch.
- Create a while loop for iterating over the file contents.
- Write/store contents of the file in the variable ch.
- Use an if condition and eof() office that is, end of the file, to ensure the compiler keeps on reading from the file if the stop is not reached.
- Use a pause statement to stop reading from the file once the end is reached.
- Impress the contents of variable ch on the console.
- End of the while body.
- Stop of the trunk of the else argument.
- Call the shut() role to close the file.
- The program must return value upon successful completion.
- End of the body of the main() role.
Summary:
- With file treatment, the output of a program can be sent and stored in a file.
- A number of operations can then exist applied to the data while in the file.
- A stream is an brainchild that represents a device where input/output operations are performed.
- A stream tin be represented as either destination or source of characters of indefinite length.
- The fstream library provides C++ programmers with methods for file handling.
- To use the library, you must include it in your program using the #include preprocessor directive.
Source: https://www.guru99.com/cpp-file-read-write-open.html
0 Response to "C++ How to Read in a File and Cout It"
Publicar un comentario